What is Array?
Arrays are used to store multiple values in a single variable. This is compared to a variable that can store only one value. Each item in an array has a number attached to it, called a numeric index, that allows you to access it. In JavaScript, arrays start at index zero and can be manipulated with various methods.
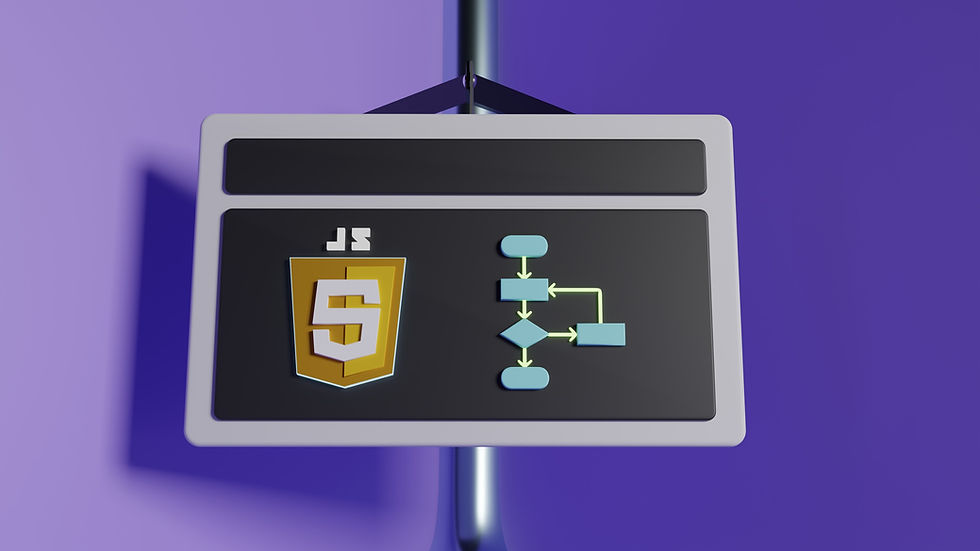
Array Methods in JavaScript
Array methods are built-in functions in JavaScript which has a special task to be performed on an array. forEach, sort, map, split, etc. are the most useful array methods. These array methods either change the original array or return a new array by modifying it.
Array Length
Before we jump to array methods let's take a look at a very important array property which is the length property.
The length property in JavaScript when applied on the array it returns the number of elements in the array. It is very frequently used when working with arrays.
Either you want to loop through the array or you want to know the number of elements in the array you can use the length property to get the number of elements in the array.
List of some Array methods in JavaScript
concat()
copyWithIn()
entries()
every()
fill()
filter()
find()
findIndex()
forEach()
Array.from()
includes()
indexOf()
isArray()
join()
lastIndexOf()
map()
push()
pop()
shift()
unshift()
reduce()
reverse()
slice()
some()
sort()
splice()
toString()
values()
Now let's look at most useful array methods in detail:
map()
filter()
find()
findIndex()
fill()
copywithin()
some()
every()
push()
pop()
shift()
unshift()
forEach()
1. JavaScript map() Array Method
The map() method in JavaScript is one of the most useful array method, it returns a new array by filling the array with the results of the callback function running for each element of the array.
You have to pass a callback function as an argument to the map() method. The callback function is called for each element of the array and the return value of the callback function is stored in the new array.
Syntax:
arr.map(callback(currentValue, index, array), thisArg)
The callback function in the map method accepts 3 arguments:
currentValue is the current element of the array.
index (optional) is the index of the current element of the array.
array (optional) is the array that is being traversed.
2. JavaScript filter() Array Method
The filter() method in JavaScript is used to create a new array with the elements of the same array if elements pass a certain condition.
The filter() methods accept a callback function as an argument.
The callback function returns true or false after checking some conditions over each and every element. If true is returned then that element is added to the new array, else discarded.
Syntax:
arr.filter(callback(currentValue, index, arr), thisArg)
The argument currentValue is necessary and index and arr are optional.
The callback function accepts three arguments:
currentValue is the current element of the array.
The index is the index of the current element.
arr is the array itself.
3. JavaScript find() Array Method
The find() method in JavaScript is returned the first value of the array elements which satisfies the provided condition.
The find() method accepts a callback function where you can define the condition.
The callback function returns true or false after checking some conditions over each and every element. If true is returned then the element is returned, else the next element is checked.
Syntax:
arr.find(callback(currentValue, index, arr), thisArg)
The callback function accepts three arguments:
currentValue is the current element of the array.
The index is the index of the current element.
arr is the array itself.
4. JavaScript findIndex() Array Method
The findIndex() method is the same as find() but it returns the index value of the element, not the value itself.
If no such element exists then returns -1.
The findIndex() method accepts a callback function where you can define the condition.
Syntax:
arr.findIndex(callback(currentValue, index, arr), thisArg)
The callback function accepts three arguments:
currentValue is the current element of the array.
The index is the index of the current element.
arr is the array itself.
5. JavaScript fill() Array Method
The fill() method in JavaScript returns a modified array by filling a specified index with the specified value.
The original array is not modified by the fill() method.
Syntax:
arr.fill(value, start, end);
The fill() method accepts 3 arguments:
value: Value to be filled
start(optional): Index from where the filling has to start. Its default value is 0.
end(optional): Index where the filling is to be stopped.
6. JavaScript copyWithIn() Array Method
The copyWithIn() method in javascript copies a part of the same array within the same calling array.
This method modifies elements of the original array but the length of the array is not modified.
Syntax:
arr.copyWithIn(targetIndex, startIndex, endIndex);
The copyWithIn() method takes three arguments:
targetIndex: It defines the target index from where a copy of the element should starts
startIndex (optional): It defines the index from where the method starts copying elements. The default value is 0.
endIndex (optional): It defines the index at which method stops copying the element. The default value is the last index.
7. JavaScript some() Array Method
The some() method in JavaScript determines whether at least one element in the array passes certain conditions by providing a callback function. If any of array element passes the test then it returns true, else returns false.
Syntax:
arr.some(callback());
8. JavaScript every() Array Method
The every() method in JavaScript executes a function for each element in the array and returns true if the function returns true for all elements.
The original array is not modified by this method.
Syntax:
arr.every(callback(currentValue, index, arr), thisArg)
You can define the callback function within the method or you can define it outside the method.
The callback function takes three arguments:
currentValue: It defines the current element in the array.
index (optional): It defines the index of the current element in the array.
arr (optional): It defines the array.
9. JavaScript push() Array Method
The push() method in javascript is used to add one or more elements to the end of the calling array.
After adding the elements to the array, the method returns the new length of the array.
Syntax:
arr.push(value1, value2, ...)
10. JavaScript pop() Array Method
The pop() method in JavaScript removes the last element from the array and returns the removed elements.
If the array is empty, the method returns undefined.
Syntax:
arr.pop()
11. JavaScript shift() Array Method
Just like the pop() method shift() method also removes the element from the array but instead of removing the last element, it removes the first element.
If the array is empty, the method returns undefined.
Syntax:
arr.shift()
12. JavaScript unShift() Array Method
Like push() method unshift() method also adds the element to the array but instead of adding it to the ending of the array, it adds it to the beginning.
This method returns the new length of the array.
Syntax:
arr.unshift(value1, value2, ...)
This method accepts 1 or more arguments.
13. JavaScript forEach() Array Method
The forEach() method in javascript is used to iterate over each and every element of the array and execute a function for all elements.
It accepts a callback function as an argument.
Syntax:
arr.forEach(callback(currentValue, index, arr), thisArg)
The callback function accepts three arguments:
currentValue is the current element of the array.
The index is the index of the current element.
arr is the array itself.
Hope you enjoyed this tutorial and learned something new. For more you can Subscribe to our YouTube channel.
Comentarios